How to Secure Your Laravel Project to Run Exclusively on a Specific Domain
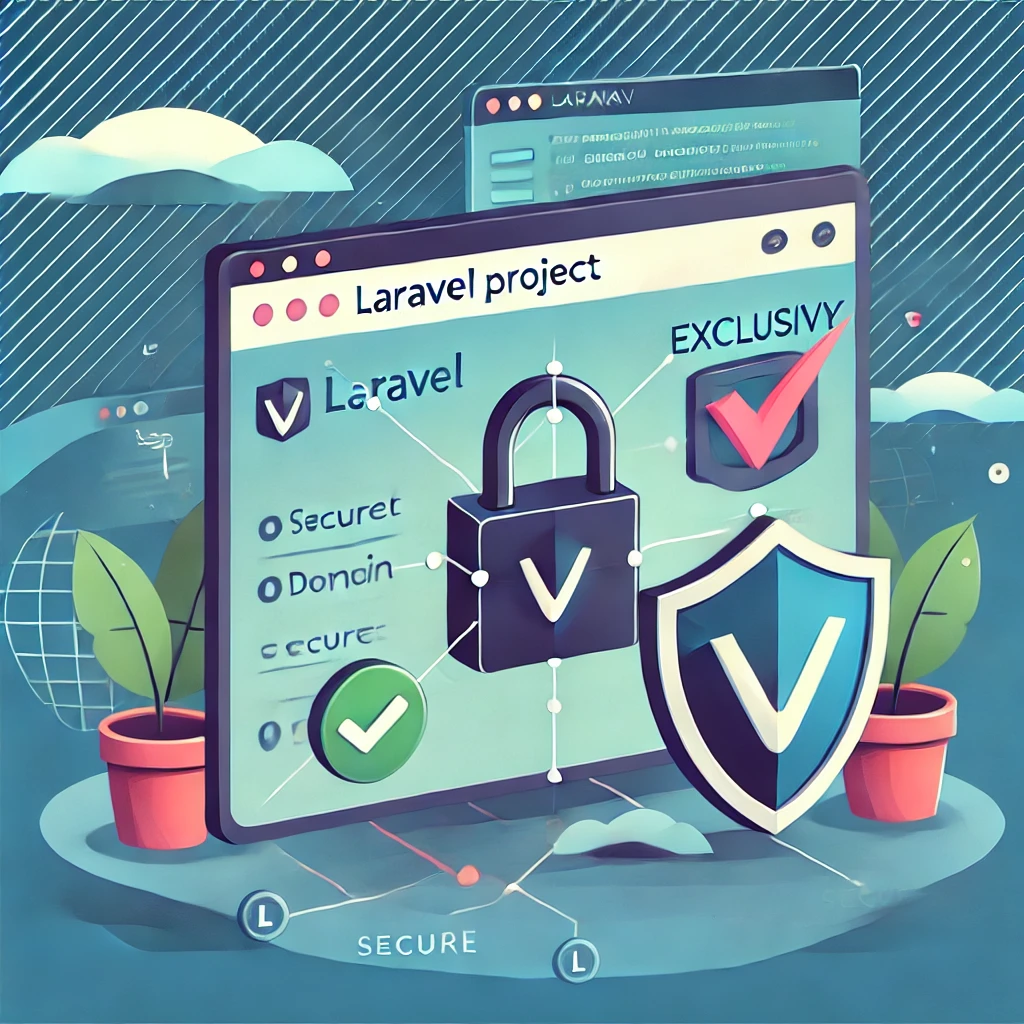
To protect your Laravel project so that it can only run on a specific domain, you can implement domain-specific validation within your application. Here are some steps to achieve this:
1. Environment Configuration
First, define the allowed domain in your .env
file. Add a new variable:
APP_NAME='eventmotoshare'
2. Middleware for Domain Check
Create a middleware to check the domain.
Create Middleware
Run the following Artisan command to create a middleware:
php artisan make:middleware DomainCheck
Middleware Logic
Edit the generated middleware file app/Http/Middleware/DomainCheck.php
to include the domain check logic:
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
class DomainCheck
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle(Request $request, Closure $next)
{
$allowedDomain = env('APP_NAME');
if ($request->getHost() !== $allowedDomain) {
abort(403, 'Unauthorized action.');
}
return $next($request);
}
}
3. Register Middleware
Register the middleware in app/Http/Kernel.php
under the web
middleware group:
protected $middlewareGroups = [
'web' => [
// Other middleware,
\App\Http\Middleware\DomainCheck::class,
],
'api' => [
// Other middleware
],
];