what is AppBar() in flutter
In Flutter, the AppBar
widget is a material design app bar that is typically placed at the top of the screen. It is used to display the title, navigation icons, and other actions related to the current screen. The AppBar
can also include a variety of widgets such as icons, text, and menus.
Constructor of AppBar class:–
AppBar(
{Key key,
Widget leading,
bool automaticallyImplyLeading: true,
Widget title,
List<Widget> actions,
double elevation,
Color shadowColor,
ShapeBorder shape,
Color backgroundColor,
Brightness brightness,
IconThemeData iconTheme,
IconThemeData actionsIconTheme,
TextTheme textTheme,
...}
)
Key Properties of Appbar Widget:–
- actions: This property takes in a list of widgets as a parameter to be displayed after the title if the AppBar is a row.
- title: This property usually takes in the main widget as a parameter to be displayed in the AppBar.
- backgroundColor: This property is used to add colors to the background of the Appbar.
- elevation: This property is used to set the z-coordinate at which to place this app bar relative to its parent.
- shape: This property is used to give shape to the Appbar and manage its shadow.
Example :–
Here’s a simple example of how to use AppBar
in a Scaffold
:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomeScreen(),
);
}
}
class HomeScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Motoshare'),
),
);
}
}
Output:–
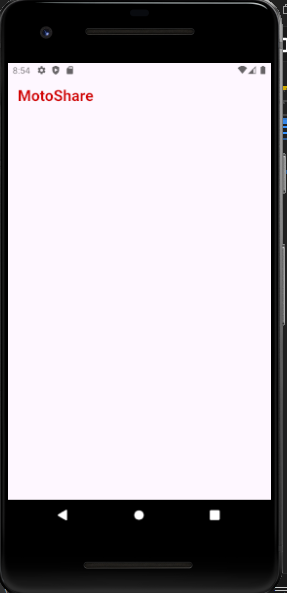